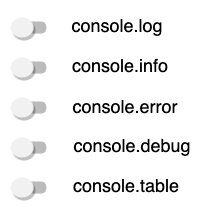
In JavaScript applications, especially on Frontend apps, we might want to disable our console.log
(or any variant of it, such as warn, info, debug, error, etc.) that we have placed for debugging throughout the application. Below are the two possible ways to do it:
1. Better Approach
Let’s create a helper.js
file that exports a log
method.
const log = (function (environment) {
if (environment === "production") {
return () => { }
}
return (...args) => {
console.log(...args)
}
})(process.env.NODE_ENV);
export {
log
}
The above logic explained:
-
We
export
the named methodlog
, which is an IIFE (Immediately Invoked Function Expression). -
To IIFE we pass an environment variable. In the above example,
process.env.NODE_ENV
is what we would pass in case of React application (built with Create React App). For Node, Express, and other applications, such as in Vue or Angular, pass their respective environment checks. -
In the IIFE, we check if the environment is “production” or not. If production, we return an empty function that would print nothing. If not production, we return a method that would pass on all the arguments to
console.log
as it is (notice the use of rest parameter and spread syntax.)
To use, import and call.
import { log } from "./helper.js";
log("Hello", 1, 2, 3, Date.now());
2. Simpler But Drastic Way
It’s usually never a good idea to modify a globally available function. Still, if you have your reasons and do not want to log anything in the production environment (or based on any other check), you can replace the console.log
method with an empty function that does nothing.
In the Node or Frontend app, place this in the file where the application starts.
if (process.env.NODE_ENV === "production") {
console.log = () => { };
}
Now throughout the application, console.log()
calls will print nothing in the production environment.
The usual console.log
for all other environments will work as expected.
The above two approaches are equally applicable to other console methods such as console.info
, console.error
, console.debug
, console.table
, console.warn
, etc.
See also
- SignatureDoesNotMatch: The request signature we calculated does not match the signature you provided. Check your key and signing method.
- Yup Date Format Validation With Moment JS
- Yup Number Validation: Allow Empty String
- Exactly Same Query Behaving Differently in Mongo Client and Mongoose
- JavaScript Unit Testing JSON Schema Validation
- Reduce JS Size With Constant Strings
- JavaScript SDK