This post discusses SDK, JavaScript/Typescript SDK, its types, benefits, and drawbacks.
What Is SDK?
SDK – Software Development Kit – is a library or a set of tools a third-party or in-house company/vendor provides for developers to integrate their services or build software. Examples are Android SDK, AWS SDK, Stripe SDK, etc.
Note that JS SDK is different from JS module, library and package.
What Is JavaScript SDK?
JavaScript (or Typescript) SDK is a library usually offered by a company for easier integration of their APIs. The library can be in one of the following environments or both:
- Browser
- Node JS
It has well-tested methods and type-support that help the developer using that third-party services in the software without worrying about the details or working of the API. Where required, the SDK takes care of interacting with the underlying broswer or operating system APIs.
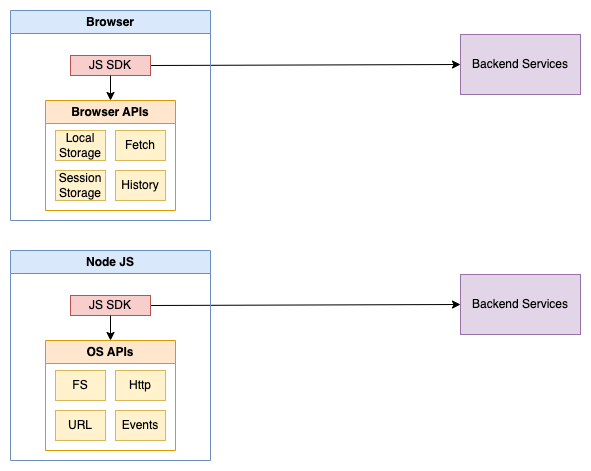
Some notable JavaScript SDKs are AWS, Facebook, and Stripe.
What Is the Difference Between UMD and NPM SDK?
Companies usually offer browser SDKs in either UMD script, NPM package, or both. Node JS applications only work with NPM packages.
In the browser environment, the library can be a UMD script or NPM package, or both. The UMD script is usable on any website/web app with a simple script tag:
<script src="https://www.sdk.example.com/"></script>
An NPM package is included in a JavaScript project with a build process.
import exampleSdk from "example-sdk";
Should an SDK Be Developed With JavaScript or Typescript?
Typescript! Because the Typescript applications using this SDK will be able to catch type errors at compile time. Even pure JavaScript applications (using the SDK with NPM) will get IDE support for code completion and type-checking because of Typescript.
What Are the Benefits of JavaScript SDK?
1- Abstraction, Lesser Lines of Code & Ease of Use
For browser or Node, a JavaScript SDK abstracts away the implementation details from developers and lets them focus on actually using the APIs. For example, the below direct API call with correct query params:
https://www.example.com/?firstName=John&lastName=Smith&greetings=HelloWorld
Can be replaced by an SDK method:
exampleSdk.sendGreetings({
greetings: "HelloWorld",
firstName: "John",
lastName: "Smith"
});
Using SDK, the developer does not need to know the underlying working of the API.
The abstraction is further helpful in maintaining development and production environments. Without the SDK, the developer has to use different APIs for multiple environments. With SDK, it’s only the env
check that needs to be set as “development” or “production” during the initialization phase of the SDK.
SDK benefits both the company and the developers because of its easier integration and requires lesser lines of code from the developer to use the APIs.
2- Documentation, Intellisense, and Versioning
Since an SDK is just a library, it comes with documentation. All the methods, parameters, and responses are well documented for the developers to use the SDK easily.
When Typescript is used to develop the SDK, the IDE (such as Visual Studio Code) assists the development process by identifying the types of parameters and return objects, in addition to code completion and numerous other features. This serves as additional documentation of the library/
The SDK is properly versioned, too. Most libraries follow the semver convention of releasing major, minor, or patch releases. This way, developers use any non-breaking versions they want to integrate into their projects.
3- Testing
The browser and Node js SDKs are tested for their environments before release. In addition to the APIs, which are tested independently, the additional layer of SDK tests ensures smooth development.
4- Better Error-Handling & Lesser Number of Bad Requests
For enterprises offering SDKs, one benefit is the lesser number of bad requests to the server, possibly saving some costs. For example, a POST call that requires greetings
to be present and in string form should request “400 bad request”. Both sets of objects sent along https://www.example.com/greetings should return 400, as they either do not have greetings
parameter present, or has non-string value in it:
{
greetings: 5
}
{
greeting: "Hello"
}
With SDK, especially built with and used in the Typescript ecosystem, this problem is caught or minimized during compilation.
import exampleSdk from "example-sdk";
exampleSdk.sendGreetings({
greetings: "Hello"
});
The result of this on the server is the removal of needless incorrect API calls.
5- More Control Over the Host Environment
On the browser, the SDK offers control over the user’s environment that doesn’t come with plain APIs. For instance, localStorage, session storage, and the cookies are accessible to the SDK, using which additional features are possible.
Similarly, the SDK version for Node JS has the option to access OS-level libraries/APIs (such as fs).
What Are the Drawbacks of JavaScript SDK?
For enterprises, the biggest drawback of releasing an SDK besides the plain APIs is the additional work. The APIs only require backend work, whereas the JS SDK needs proper handling of the respective environments (browser, Node JS), browser compatibility, release notes, versioning, and testing. The ease of use for developers means more work on the company’s side.
Moreover, in the case of the backend, JavaScript SDK might be one of many SDKs the company has to release. PHP, .Net, Ruby, Go, and many other vibrant backend communities would like to have the company’s SDK in their programming language. A language-agnostic set of APIs would work with all backend technologies.
From the developer’s perspective, integrating APIs directly is probably more size efficient. Having another NPM package adds to the size of an already bloated build. However, this is not much of a problem with Node JS packages, as backend application size is usually not a consideration.
See also
- SignatureDoesNotMatch: The request signature we calculated does not match the signature you provided. Check your key and signing method.
- Yup Date Format Validation With Moment JS
- Yup Number Validation: Allow Empty String
- Exactly Same Query Behaving Differently in Mongo Client and Mongoose
- JavaScript Unit Testing JSON Schema Validation
- Reduce JS Size With Constant Strings
- JavaScript: Find if the String Has a Particular Case