In continuation of my previous post Convert HTML to PDF in Node JS without headless browser, I will show you how can we can fetch the images from URL, convert them into base64 string, and populate them in the HTML that is then converted into PDF.
The Code
First, install the required packages:
npm i html-to-pdfmake jsdom pdfmake axios
Then run the following code script with node to download the example-image.pdf
file that contains the image:
(You can also get the code from Github repo.)
const pdfMake = require("pdfmake/build/pdfmake");
const pdfFonts = require("pdfmake/build/vfs_fonts");
pdfMake.vfs = pdfFonts.pdfMake.vfs;
const fs = require("fs");
const jsdom = require("jsdom");
const { JSDOM } = jsdom;
const { window } = new JSDOM("");
const htmlToPdfMake = require("html-to-pdfmake");
const axios = require("axios");
// https://bobbyhadz.com/blog/convert-image-to-base64-using-node#convert-an-image-url-to-base64-in-nodejs-using-axios
async function imageUrlToBase64(url) {
try {
const response = await axios.get(url, {
responseType: "arraybuffer",
});
const contentType = response.headers["content-type"];
const base64String = `data:${contentType};base64,${Buffer.from(
response.data
).toString("base64")}`;
return base64String;
} catch (err) {
console.log(err);
}
}
const imageUrl = "https://loremflickr.com/400/400";
let HTML = `
<div>
<h1>This below image is downloaded from <a href="https://loremflickr.com" target="_blank">LoremFlickr</a> on Node js with axios and converted to PDF!</h1>
<img src="${imageUrl}" alt="LoremFlickr Random Image" width="400" height="400">
</div>
`;
async function start() {
HTML = HTML.replace(
imageUrl,
await imageUrlToBase64(imageUrl)
);
const html = htmlToPdfMake(HTML, {
window
});
const docDefinition = {
content: [html],
// pageOrientation: "landscape",
};
const pdfDocGenerator = pdfMake.createPdf(docDefinition);
pdfDocGenerator.getBuffer(function (buffer) {
fs.writeFileSync("example-image.pdf", buffer);
});
}
start();
Note the imageUrlToBase64
method copied from bobbyhadz blog. It downloads the image from URL into buffer and then gives out a base64 string.
Later on, we replace the image URL in the HTML file with base64 string, and create a pdf with the help of pdfMake.createPdf
.
The example uses LoremFlickr link which gives a new image each time. The generated PDF will look something like this:
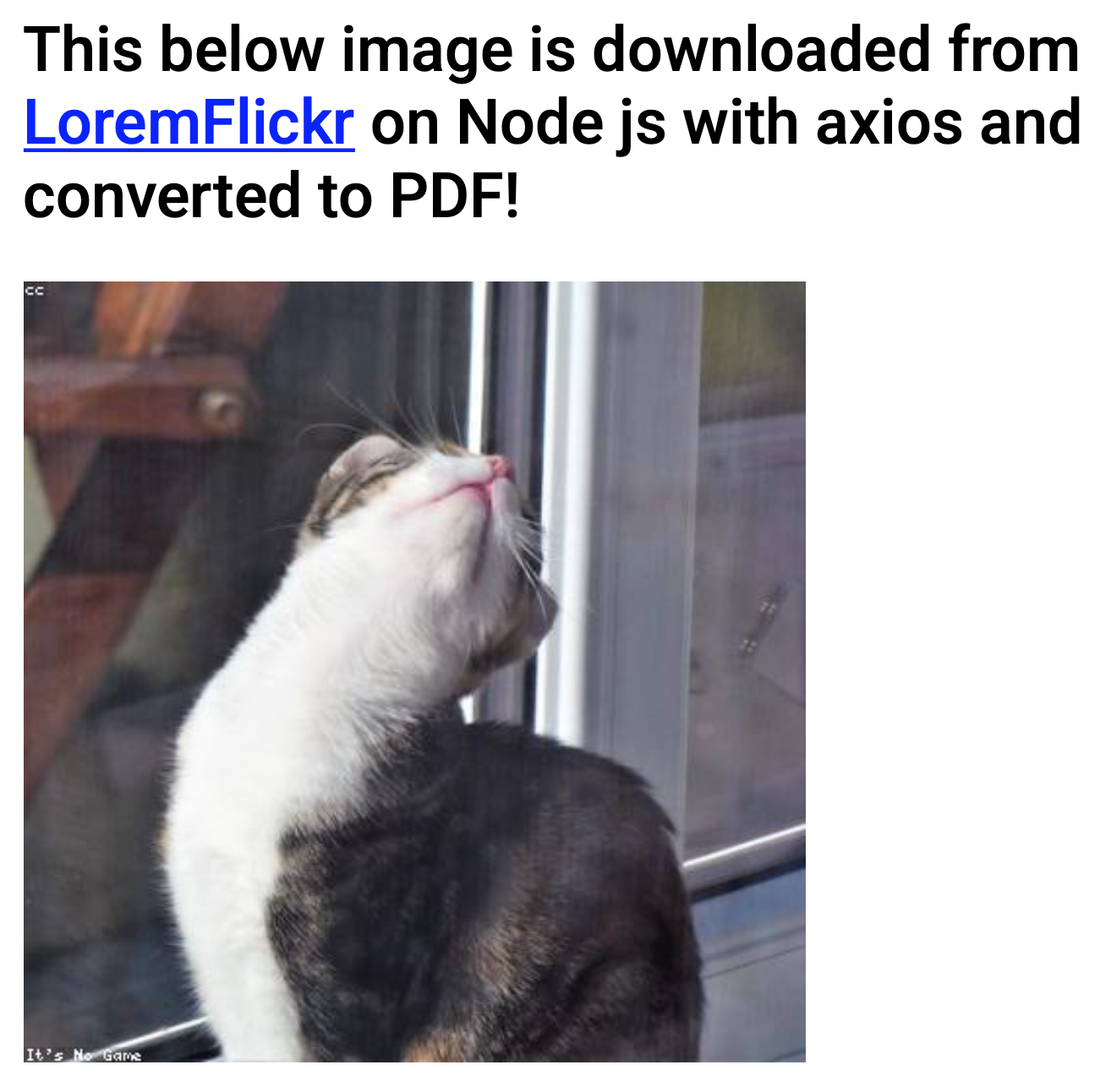
See also
- Node JS Mongo Client for Atlas Data API
- SignatureDoesNotMatch: The request signature we calculated does not match the signature you provided. Check your key and signing method.
- Exactly Same Query Behaving Differently in Mongo Client and Mongoose
- MongoDB Single Update Query to Change the Field Name in All Matching Documents of the Collection
- AWS Layer: Generate nodejs Zip Layer File Based on the Lambda's Dependencies
- Convert HTML to PDF in Nodejs
- JavaScript Rollbar: Unknown Unhandled Rejection Error Getting Reason From Event