To convert an HTML to PDF in the backend, you usually need a headless browser like Puppeteer or phantomjs. The downside of headless browsers is that they are resource intensive. If you need to convert an HTML in Nodejs, is there a way to do so without them? The answer is yes but with a caveat: The HTML should be relatively simple, and you will only have a few options to style the document.
Below, let’s see how we can achieve that.
The Code
Install these libraries with npm or yarn:
npm i html-to-pdfmake jsdom pdfmake
Now run the self-explanatory code below to generate a pdf that has a table of famous companies. You should get companies.pdf
file in the directory where you run the script:
(You can also get the code from Github repo.)
const pdfMake = require("pdfmake/build/pdfmake");
const pdfFonts = require("pdfmake/build/vfs_fonts");
pdfMake.vfs = pdfFonts.pdfMake.vfs;
const fs = require("fs");
const jsdom = require("jsdom");
const { JSDOM } = jsdom;
const { window } = new JSDOM("");
const htmlToPdfMake = require("html-to-pdfmake");
const HTML = `
<div>
<h1>World Companies</h1>
<p>Following is the data of some notable companies:</p>
<table>
<tr>
<th>Company</th>
<th>Headquarters</th>
<th>Country</th>
</tr>
<tr>
<td>Google</td>
<td>Mountain View, California</td>
<td>United States</td>
</tr>
<tr>
<td>Facebook</td>
<td>Menlo Park, California</td>
<td>United States</td>
</tr>
<tr>
<td>Bosch</td>
<td>Gerlingen</td>
<td>Germany</td>
</tr>
<tr>
<td>Honda</td>
<td>Minato City, Tokyo</td>
<td>Japan</td>
</tr>
<tr>
<td>Tata</td>
<td>Mumbai</td>
<td>India</td>
</tr>
</table>
</div>
`;
const html = htmlToPdfMake(HTML, {
window
});
const docDefinition = {
content: [html],
// pageOrientation: "landscape",
styles: {
"html-h1": {
color: "#003366",
background: "white",
},
},
};
const pdfDocGenerator = pdfMake.createPdf(docDefinition);
pdfDocGenerator.getBuffer(function (buffer) {
fs.writeFileSync("companies.pdf", buffer);
});
The pdf will look something like this:
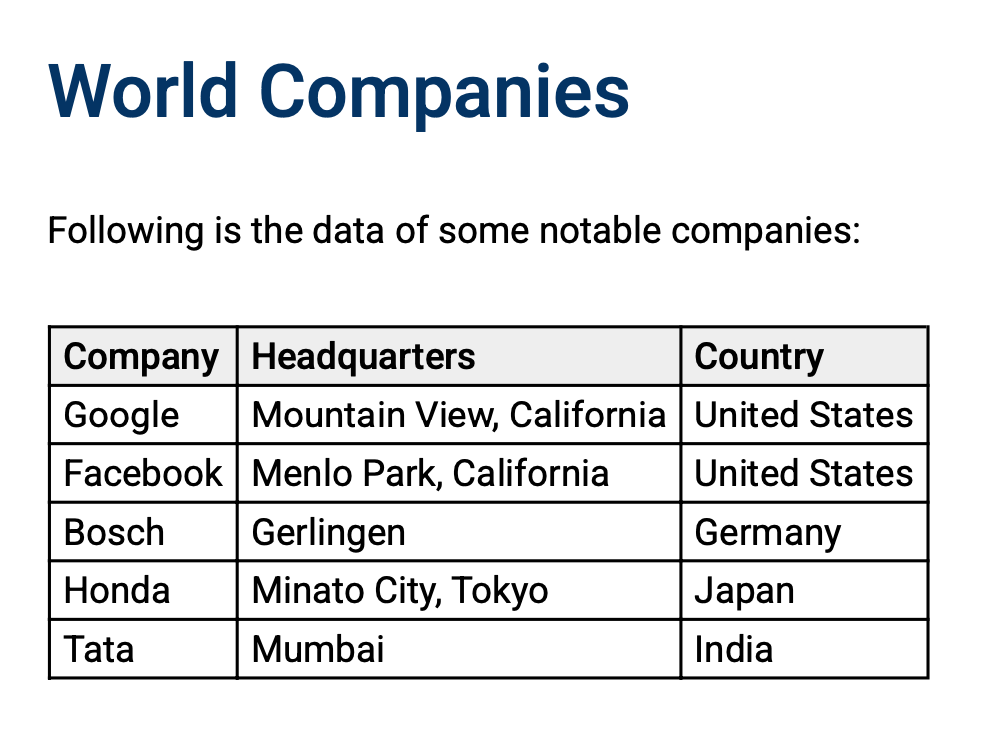
Notice the color styling of h1. To get a landscape pdf, pass pageOrientation: "landscape"
in the docDefinition (commented).
To know how to include images from url in HTML to PDF generation check In Node JS HTML to PDF conversion, Populate Image URLs
See also
- Node JS Mongo Client for Atlas Data API
- SignatureDoesNotMatch: The request signature we calculated does not match the signature you provided. Check your key and signing method.
- Exactly Same Query Behaving Differently in Mongo Client and Mongoose
- MongoDB Single Update Query to Change the Field Name in All Matching Documents of the Collection
- AWS Layer: Generate nodejs Zip Layer File Based on the Lambda's Dependencies
- In Node JS HTML to PDF conversion, Populate Images From URLs
- JavaScript Rollbar: Unknown Unhandled Rejection Error Getting Reason From Event