JavaScript Inheritance and Polymorphism in React JS Application
Serialize and Deserialize JavaScript Class Objects In Front End Applications
The drawback of using JavaScript classes in your frontend application is that we cannot pass it to or receive it from the backend as class object. We need to serialize and deserialize it.
[Read More]JavaScript Get Information of Week Days Between Two Dates
With and Without Moment JS
What Are The Use Cases of Lodash Chunk method?
JavaScript Flatten Deeply Nested Array of Objects Into Single Level Array
Using plain JavaScript, and lodash's flatMapDeep method.
JavaScript Find Path of Key in Deeply Nested Object or Array
Key Path Finder Using Depth First Search (DFS)
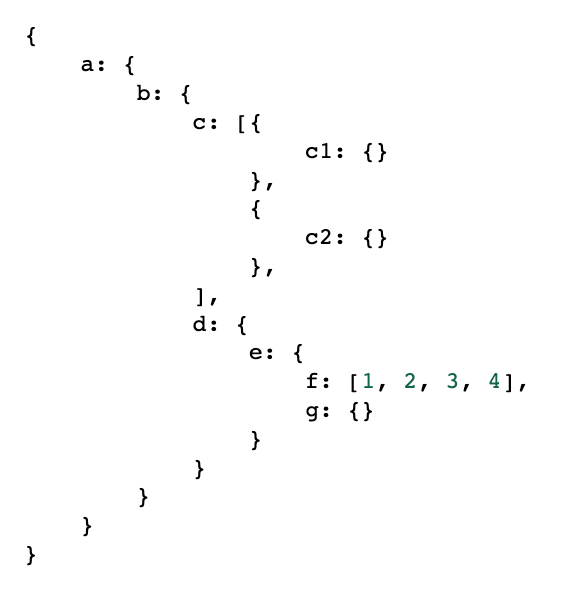
The following two codes go through the whole object, an array of objects, or a collection of both to get the path to a particular key. There are two versions: first gets the path to the key only, and second gets the path where a key has the given value.
[Read More]JavaScript Check if Key Exists in Deeply Nested Object or Array of Objects, Without Knowing the Path
Depth First Search (DFS) for Key Verification in an Object
The following JavaScript code recursively goes through the whole object, an array of objects, or a collection of both to verify if the given key exists somewhere down the object. It does not require the path to the key in advance.
A similar recursive solution as below can also be used to modify all the keys of a deeply nested object or array of objects.
[Read More]